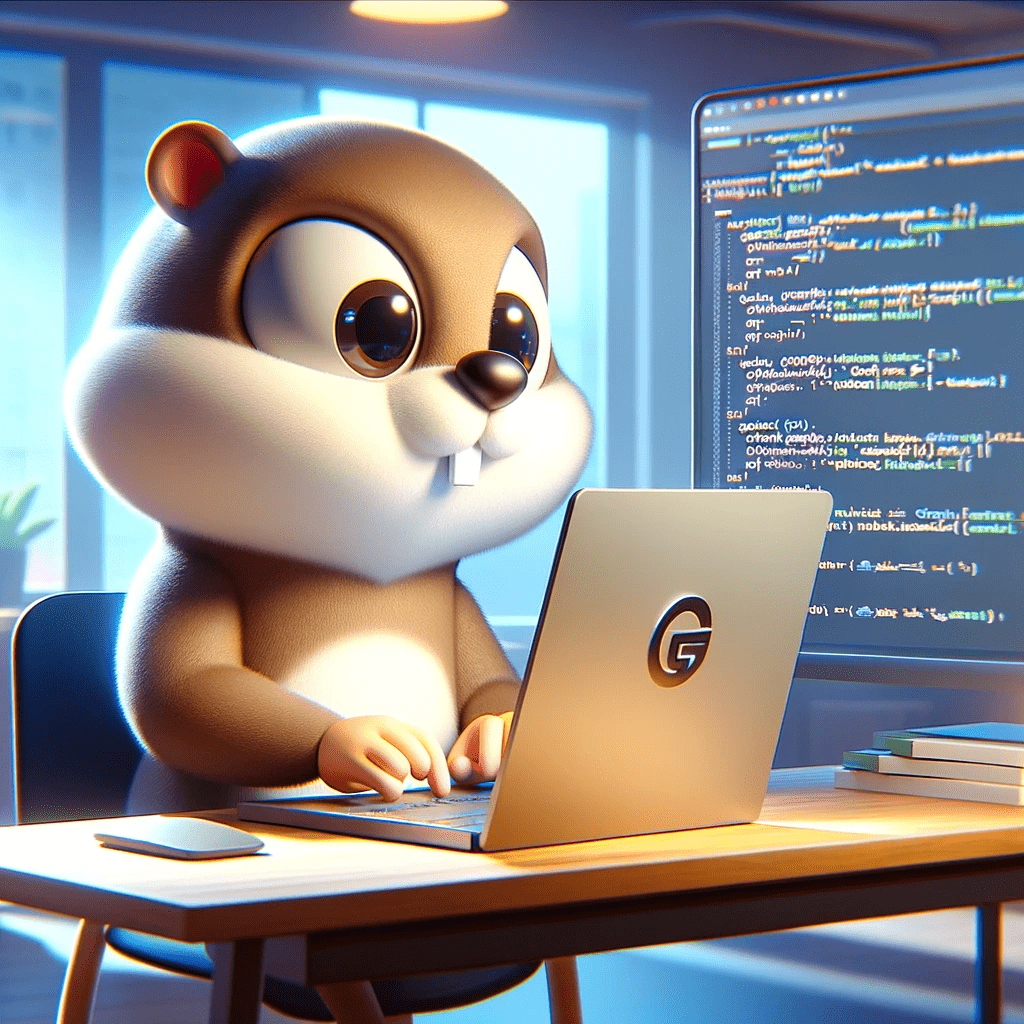
In the dynamic world of web development, the search for efficient, robust, and scalable programming languages is constant. Golang, also known as Go, has emerged as a strong contender, offering simplicity, high performance, and concurrency support. As a web development agency, AZdev recognizes the potential of Golang in building modern web applications. This article dives into how Golang can be leveraged in web development, supported by clever code samples.
Introduction to Golang
Developed by Google, Golang is an open-source programming language that makes it easy to build simple, reliable, and efficient software. It’s known for its speed, which comes from its compiled nature, as well as its straightforward syntax and powerful standard library.
Why Choose Golang for Web Development?
Golang offers several advantages for web developers:
- Performance: As a compiled language, Go runs incredibly fast, which is crucial for web services that handle many requests simultaneously.
- Concurrency: Go’s built-in support for concurrency with Goroutines and Channels makes it ideal for handling multiple tasks simultaneously, a common requirement in web services.
- Simplicity and Readability: Go’s syntax is clean and easy to understand, making the codebase more maintainable.
- Robust Standard Library: Go’s standard library comes packed with utilities that make web development smoother, including an HTTP server and tools for JSON encoding and decoding.
Creating a Basic Web Server in Go
Let’s start with a basic example of a web server in Go. This sample demonstrates how to set up a simple HTTP server.
package main
import (
"fmt"
"net/http"
)
func main() {
http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, "Hello, you've requested: %s\n", r.URL.Path)
})
http.ListenAndServe(":8080", nil)
}
In this example, we import the necessary packages, set up a route handler, and start a server that listens on port 8080. When you navigate to http://localhost:8080
, you’ll see a greeting and the requested URL path.
Handling Concurrency with Goroutines
A significant feature of Go is its ability to handle multiple requests concurrently. Here’s a simple example to illustrate this:
package main
import (
"fmt"
"net/http"
"time"
)
func responseHandler(w http.ResponseWriter, r *http.Request) {
time.Sleep(2 * time.Second) // Simulate time-consuming task
fmt.Fprintf(w, "Request processed")
}
func main() {
http.HandleFunc("/", responseHandler)
http.ListenAndServe(":8080", nil)
}
Each request to the server takes 2 seconds to process. With Go’s concurrency model, the server can handle multiple requests at the same time, each running in its own Goroutine.
Utilizing Go Templates for Dynamic Web Pages
Golang also simplifies the creation of dynamic web pages using templates. Here’s a basic example:
package main
import (
"html/template"
"net/http"
)
type PageVariables struct {
Title string
Body string
}
func main() {
http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) {
pageVariables := PageVariables{
Title: "Golang Web Development",
Body: "This is a sample web page!",
}
t, _ := template.ParseFiles("template.html")
t.Execute(w, pageVariables)
})
http.ListenAndServe(":8080", nil)
}
In this code, template.html
is a HTML file with Go template syntax. The server fills this template with data from PageVariables
struct and serves a dynamic web page.
Building RESTful APIs with Go
Golang is also excellent for building RESTful APIs. Here’s a simplified example of a CRUD API:
package main
import (
"encoding/json"
"net/http"
)
type Item struct {
ID string `json:"id"`
Name string `json:"name"`
}
var items = []Item{
{ID: "1", Name: "Item 1"},
{ID: "2", Name: "Item 2"},
}
func getItems(w http.ResponseWriter, r *http.Request) {
json.NewEncoder(w).Encode(items)
}
func main() {
http.HandleFunc("/items", getItems)
http.ListenAndServe(":8080", nil)
}
This code sets up a simple API that returns a list of items in JSON format.
Conclusion
Golang’s simplicity, coupled with its performance and concurrency features, makes it an increasingly popular choice for web development. Whether it’s creating a basic web server, handling multiple requests concurrently, generating dynamic web pages, or building RESTful APIs, Go’s robust standard library and