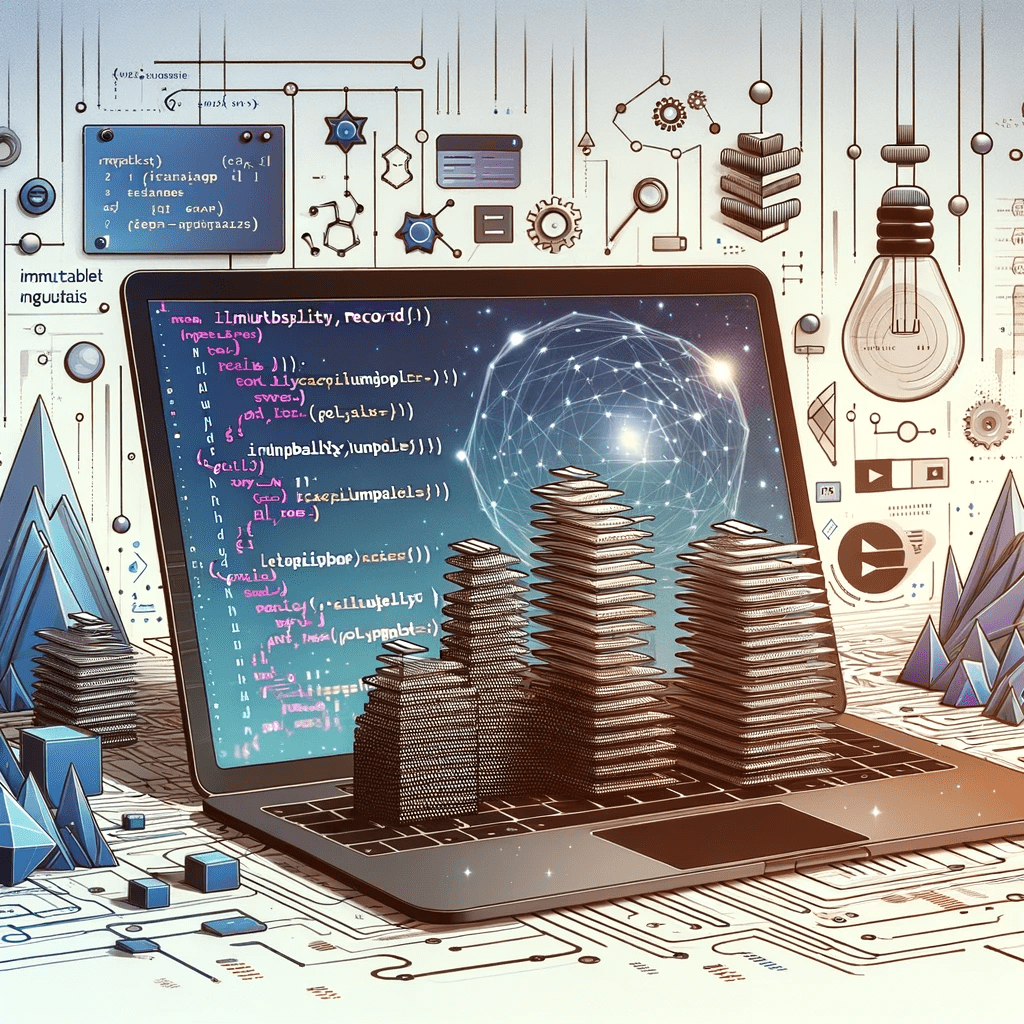
In the ever-evolving world of JavaScript, new features and proposals continuously shape and redefine the landscape of web development. Among these, the “Records and Tuples” proposal stands out, introducing immutable data structures that promise to enhance code efficiency and clarity. This article delves into the nuts and bolts of Records and Tuples in JavaScript, unraveling their potential impact on modern web development.
Understanding Records and Tuples
Records and Tuples are proposed as new primitive types in JavaScript, bringing the concept of deep immutability to the language. While Objects and Arrays are mutable data types, Records and Tuples are their immutable counterparts.
- Records: An immutable collection of keyed values, similar to an Object but cannot be altered once created.
- Tuples: An immutable list of values, similar to an Array, with a fixed length and sequence of data types.
The Concept of Deep Immutability
Deep immutability means that not only is the Record or Tuple itself immutable, but all of its elements are also immutable. This characteristic is a game-changer for ensuring data integrity and predictability in code.
Benefits of Using Records and Tuples
Using immutable data structures like Records and Tuples in JavaScript can lead to:
- Enhanced Code Safety: Prevents accidental modifications of data, which can lead to bugs.
- Performance Optimization: Enables efficient data handling and comparison, as immutable structures can be easily optimized by JavaScript engines.
- Improved Developer Experience: Offers clear semantics for data structures that shouldn’t change, leading to more readable and maintainable code.
Records in Depth
Records are like Objects, but they are completely immutable. They are denoted by a double curly brace #{}
syntax.
Creating and Using Records
const bookRecord = #{
title: "1984",
author: "George Orwell"
};
// Attempting to modify will throw an error
// bookRecord.author = "Someone else"; // TypeError: Cannot assign to read-only property
Comparing Records
One of the key features of Records is that they can be compared by value rather than by reference.
const record1 = #{ a: 1, b: 2 };
const record2 = #{ a: 1, b: 2 };
console.log(record1 === record2); // true
Use Case: Storing Configuration
Records are perfect for storing configuration objects that you don’t want to be accidentally modified.
Tuples in Depth
Tuples are immutable lists and are denoted by the double square bracket #[]
syntax.
Creating and Using Tuples
const colorTuple = #['red', 'green', 'blue'];
// Trying to modify a tuple will result in an error
// colorTuple[0] = 'yellow'; // TypeError: Cannot assign to read-only property
Comparing Tuples
Like Records, Tuples also offer value-based comparison.
const tuple1 = #[1, 2, 3];
const tuple2 = #[1,
2, 3];
console.log(tuple1 === tuple2); // true
This comparison feature is particularly useful in scenarios where you need to compare the content of two sequences, such as in React’s component state management.
Use Case: Function Arguments
Tuples are ideal for grouping a fixed number of elements, making them excellent for function arguments where the order and type of elements are fixed.
function drawLine(startPoint, endPoint) {
// startPoint and endPoint are tuples
console.log(`Drawing line from ${startPoint} to ${endPoint}`);
}
drawLine(#[x1, y1], #[x2, y2]);
Practical Applications
The introduction of Records and Tuples can significantly impact the way we handle data in JavaScript. For instance, in state management, immutable structures can lead to more predictable state changes and easier bug tracking. In functional programming, they ensure data integrity throughout transformations and operations.
Potential Limitations and Considerations
While Records and Tuples bring many benefits, there are considerations to keep in mind:
- Browser and Environment Support: As a Stage 2 proposal (as of writing), it’s not yet widely supported in all JavaScript environments.
- Learning Curve: Developers accustomed to working with mutable types may need time to adapt to immutable structures.
Conclusion
The proposal for Records and Tuples in JavaScript marks a significant step towards more robust, efficient, and predictable coding practices. By providing immutable data structures, JavaScript continues to evolve, addressing the needs of complex applications and aligning with functional programming paradigms. As we await their full integration into the language, it’s an exciting time to experiment with these features and envision how they will shape the future of JavaScript development. For a web development agency like AZdev, staying ahead of such innovations is key to delivering cutting-edge solutions.